Hello,
We apologize for the issue you are facing with retrieving meta tags via the Rank Math API in your Headless CMS setup. Thank you for raising this concern.
To help you implement this functionality correctly, here are the steps you should follow:
Step 1: Enable Headless CMS Support
Make sure that you have enabled the Headless CMS Support option in Rank Math. You can do this by navigating to Rank Math SEO → General Settings → Others and enabling the option. Don’t forget to save the changes.
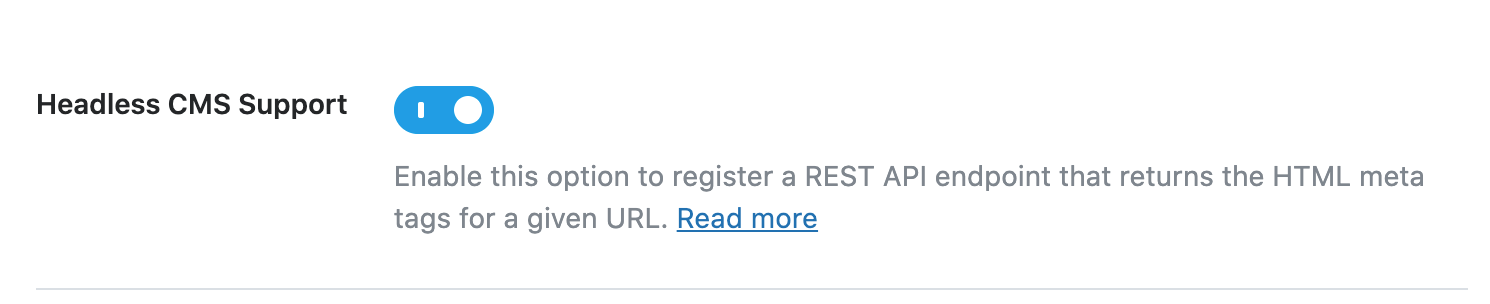
Step 2: Make a GET Request to the REST API
You need to send a GET request to the Rank Math REST API endpoint using the following syntax:
https://your-domain.com/wp-json/rankmath/v1/getHead?url=https://your-domain.com/hello-world?url=https://your-domain.com/hello-world
Replace your-domain.com
with your actual domain and `https://your-domain.com/wp-json/rankmath/v1/getHead?url=https://your-domain.com/hello-world?url=https://your-domain.com/hello-world with the URL of the page for which you want to retrieve the meta tags.
Step 3: Whitelist the REST API Endpoint
If you are unable to access the Rank Math REST endpoint, it might be due to a security plugin blocking it. Ensure that you whitelist the following endpoint in your firewall:
https://your-domain.com/wp-json/rankmath/v1/getHead?url=https://your-domain.com/hello-world
You can refer to our tutorials on how to whitelist Rank Math’s REST API endpoints if you are using plugins like Wordfence or Cloudflare.
Step 4: Handle Errors
If you encounter errors while making requests, here are some common issues:
- No Route Was Found Matching the URL and Request Method: This indicates that Headless CMS support is not enabled.
- Invalid Parameter(s) URL: Ensure that you are using a complete URL and not just a slug.
- Missing Parameter(s) URL: Make sure that your request includes the URL parameter as described.
Example of Fetching Meta Tags in React
Here’s a simple example of how you might implement this in your React frontend:
import React, { useEffect, useState } from 'react';
const MetaTagsFetcher = ({ pageUrl }) => {
const [metaTags, setMetaTags] = useState(null);
useEffect(() => {
const fetchMetaTags = async () => {
const response = await fetch(`https://your-domain.com/wp-json/rankmath/v1/getHead?url=https://your-domain.com/hello-world?url=${pageUrl}`);
const data = await response.json();
if (data.success) {
setMetaTags(data.head);
} else {
console.error('Error fetching meta tags:', data);
}
};
fetchMetaTags();
}, [pageUrl]);
return ;
};
Replace pageUrl
with the actual URL of the page you want to fetch meta tags for.
If you continue to experience issues, please reply to this ticket, and a human agent from the Rank Math Support team will assist you further.
We hope this helps you set up your Headless CMS with Rank Math successfully!